What is NodeJS and How It Works?
Table of Contents
Node.js, which Ryan Dahl first released in 2009, has completely changed web development by allowing JavaScript, a single programming language, to be used for both client-side and server-side scripting. Because Node.js is based on the highly performant and efficient V8 JavaScript engine, it is especially well-suited for real-time and scalable network applications. It employs an event-driven, non-blocking I/O approach.
The process by which developers create server-side apps has been transformed by Node.js. It is a helpful tool for developing expansive, effective online applications because of its distinctive non-blocking, event-driven architecture. In this article, we will look into the NodeJS foundation and its inner workings.
Is NodeJS a Programming Language?
Contrary to popular belief, Node.js is not a programming language. In actuality, it is a runtime environment that allows JavaScript code to be executed outside of a web browser. Node.js gives JavaScript, the computer language frequently used to produce dynamic and interactive online content, server-side capabilities. JavaScript is used to manipulate the DOM (document object model) or website page that is loaded in the browser, like popups and models. Basically, JavaScript is a language that runs in the browser and allows you to interact with the page after it is loaded.
NodeJS is basically a different version of JavaScript; you can say it builds on JavaScript.
JavaScript Run-time Environment:
Understanding the concept of a runtime environment is essential to understanding Node.js. The infrastructure and services needed for a programming language to execute code are provided by a runtime environment. Using the potent V8 JavaScript engine, Node.js offers a means for JavaScript to execute on the server side.
JavaScript code can be executed outside of a web browser thanks to the runtime environment that NodeJS offers. It enables you to run JavaScript code not only on the server but also on any machine. This means we can use NodeJS code to run outside of the browser. The JavaScript engine, fundamental libraries, and APIs are a few examples of the different parts that make up a runtime environment. Based on the V8 engine, Node.js integrates these components to give JavaScript more functionality and the ability to do a wide range of tasks, such as reading from and writing to file systems, managing data with Databases, generating business logic, responding network requests, and more. Node.js’s runtime environment simplifies server-side development by allowing developers to use a single language (JavaScript) for both client-side and server-side scripting. The development process is streamlined and developers’ cognitive strain is lessened by this unity.
Diagram to show the Flow of NodeJS:
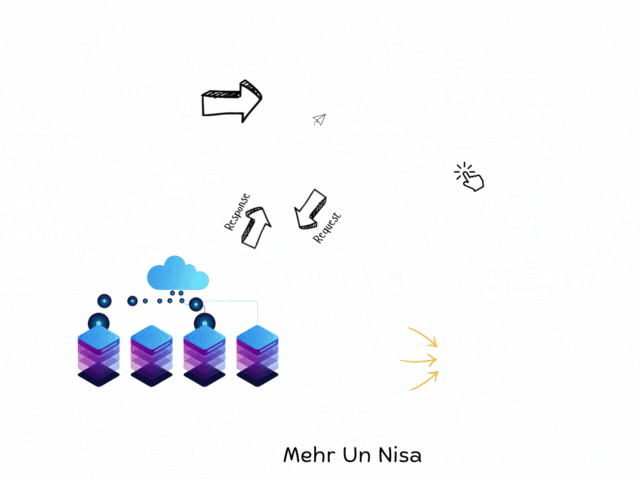
Node.js has greatly increased the possibilities of JavaScript outside of the browser. JavaScript has historically been used mostly for client-side programming, which adds dynamic, interactive features to web sites and allows them to react instantly to user input inside the browser. But with Node.js, this scenario is drastically altered. Node.js turns JavaScript into a full-stack development tool by offering a runtime environment for server-side JavaScript code execution. With this change, programmers can use a single programming language to design whole web apps.
Programmers can create dynamic web pages before they are transmitted to the user’s browser by using Node.js to do server-side scripting. Historically, only programming languages like PHP, Python, or Ruby were capable of doing this. With its event-driven, non-blocking I/O approach, Node.js is based on the high-performance V8 JavaScript engines from Google Chrome technology. Applications that need for real-time, concurrent processing, such chat programs, online games, or live data feeds, are especially well suited for this architecture.
Now that JavaScript can be used to build both front-end and back-end code, developers may streamline their workflow, minimize context switching, and create a more cohesive codebase. With just one language to learn and use, this unification not only speeds up the onboarding process for new engineers but also streamlines development. Because of this, Node.js is becoming a popular choice for modern web development, fostering innovation and efficiency in the creation of scalable, effective web applications.
Is NodeJS a Framework:
Another widespread misperception is that Node.js is a framework, even though this is untrue. For the avoidance of doubt, a framework is an organized set of pre-written code that serves as the basis for creating applications. In order to expedite the development process and uphold best practices, frameworks frequently come with predefined templates, tools, and conventions. By offering reusable components and a standard architecture, they are made to facilitate the development of applications by developers. Node.js is a runtime environment, in comparison. The infrastructure and services required to run code written in a particular programming language—in this example, JavaScript—are provided by a runtime environment. JavaScript may now operate on the server side, independent of a web browser, thanks to Node.js. It offers functions that are not possible in the typical browser-based JavaScript environment, like file system access, network connectivity, and process management. Node.js is not a framework in and of itself, but it does have a robust ecosystem of frameworks and libraries that make development easier and more enjoyable. NodeJS runs on a server and has access to the server’s file systems, networks, and other resources. It does not have access to the DOM because there is no web page to manipulate; instead, it interacts with the OS, database, and other servers.
NodeJS Frameworks Lists:
Node.js has a robust ecosystem of frameworks, including Express.js, Koa.js, and Hapi.js. These frameworks give programmers access to libraries and tools that make creating web apps, APIs, and other applications faster. They take care of routine duties like templating, middleware administration, and routing so that developers can concentrate on creating the essential features of their apps.
- Express.js: Popular web application framework Express.js, which is based on Node.js, offers powerful functionality for creating both web and mobile applications. It makes middleware management, request and response processing, routing, and other related activities simpler, freeing developers to concentrate more on application logic and less on boilerplate code.
- Koa.js: The developers of Express also created Koa.js, a more contemporary and modular method. It handles middleware with async methods, which improves readability and maintainability of the code. Koa wants to provide a more compact, expressive, and reliable base for APIs and web apps.
- Hapi.js: Another strong framework with an extensive feature set right out of the box is Hapi.js. By prioritizing configuration over code, it helps developers create apps with fewer superfluous code.
V8 Engine JavaScript:
Node.js is built on the V8 engine, a Google-developed component. Node.js is built around the V8 JavaScript engine, which is an open-source engine developed by Google. V8 is very quick and effective since it compiles JavaScript directly into native machine code. One of the reasons Node.js is a good choice for developing scalable network applications is this performance improvement.
V8 takes the JavaScript code and compiles it to machine code, same happens on our computer. NodeJS takes the V8 codebase and adds the certain features like working with your local files systems, opening files, reading files and deleting files etc.
Diagram of V8 Engine Working with NodeJS:
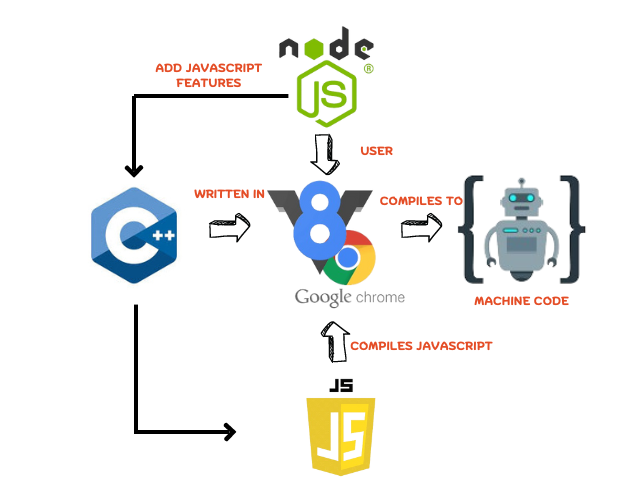
What is the relationship between Node.js and V8?
V8 engine:
- Developed by Google: Google created the open-source V8 JavaScript and Web Assembly engine. It is used to run JavaScript code in Google Chrome and other Chromium-based browsers.
- High Performance: Because V8 is built for great efficiency, it executes JavaScript quicker than if it were interpreted line by line by first compiling it to machine code.
- Standalone Component: Despite being a stand-alone component, V8 is integrated to enable JavaScript execution into other programs, such as web browsers and Node.js.
Node.js:
- Built on V8: The V8 engine, which powers Node.js, is designed to execute JavaScript at a high speed. A runtime environment with libraries and APIs for server-side development is added by Node.js.
- Runtime Environment: Apart from V8, Node.js comes with a number of built-in modules (such as fs, http, etc.) that offer more capabilities for managing file systems, network connections, and other server-side operations.
Node.js and V8 Engine
JavaScript code is executed by Node.js using the V8 engine, which it employs to run independently of web browsers.
Browser Independence:
- Standalone Execution: Node.js can run JavaScript code without the need for a browser. Rather, it executes JavaScript in any environment that has Node.js installed or on the server side.
- Cross-Platform: JavaScript code created specifically for Node.js can execute on any system that has Node.js installed, independent of the underlying operating system or browser, because Node.js comes with its own V8 engine.
The V8 engine is included in the installation package when you install Node.js locally. The V8 engine is part of the Node.js installation package. This indicates that V8 comes pre-installed with Node.js, so you don’t need to install it separately. The V8 engine is installed automatically when you download and install Node.js from the official website or using a package manager (such as npm for JavaScript packages).
The V8 engine is included in the installation package when you install Node.js locally. The V8 engine is part of the Node.js installation package. This indicates that V8 comes pre-installed with Node.js, so you don’t need to install it separately. The V8 engine is installed automatically when you download and install Node.js from the official website or using a package manager (such as npm for JavaScript packages). Node.js serves as a bridge, running JavaScript on V8 and offering further features via its own built-in modules and APIs.
For Example:
The JavaScript engine of the specific browser (V8, Spider Monkey, JavaScript Core, or Chakra) is utilized to run the code when you run JavaScript in that browser.
Browser Environment (Chrome): Uses V8 engine.
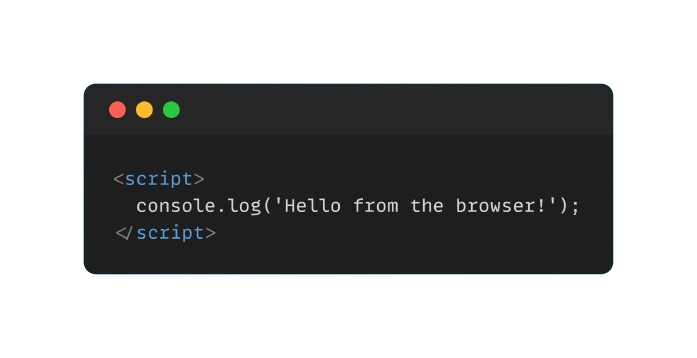
Node.js Environment: Uses V8 engine bundled with Node.js.
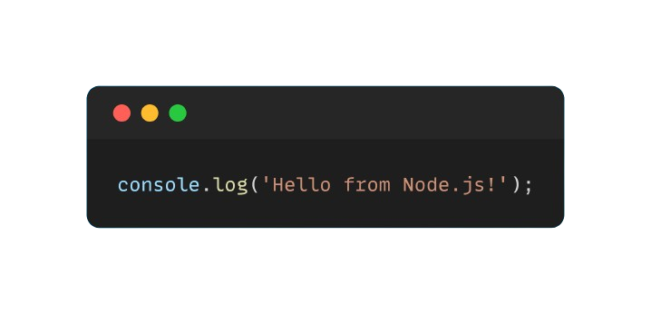
Run in the terminal:
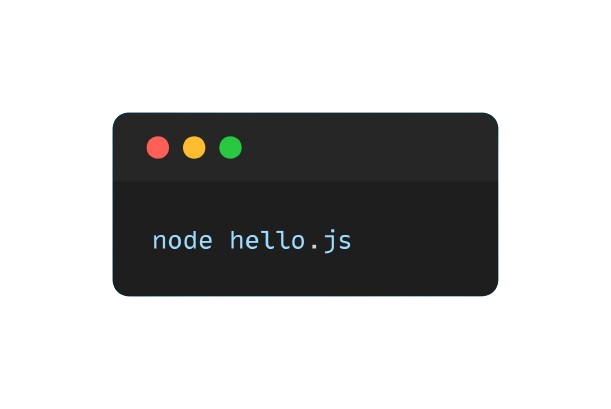
Conclusion:
JavaScript code is executed on the server side by Node.js using the V8 engine, which runs independently of browsers. This creates a unified language environment by enabling JavaScript to be used for both client-side (browser) and server-side (Node.js) development.
Without depending on the JavaScript engine of any browser, Node.js executes JavaScript code using its own integrated V8 engine. As a result, JavaScript code developed for Node.js can run on any platform that supports Node.js, regardless of the browser used.
Advantages of NodeJS:
- More than a server-side language: you can use Node.js in utility scripts and build tools like the build processes of other languages, frameworks, etc.
- Helps in Writing a server: with the help of Node.js, you can write the server yourself. It helps to write the server itself.
As in PHP, you have extra tools like Apache or NGINZ that run the server, listen to incoming requests, and then execute the PHP codes. NodeJS does both to listen to the incoming requests and give the responses.
- Asynchronous, Event-driven Architecture: The asynchronous, event-driven architecture of Node.js is its key feature. In contrast to conventional server-side environments, which depend on synchronous processing, Node.js uses an event-driven, non-blocking architecture. Because of its ability to effectively manage several concurrent connections, this makes it especially suitable for developing highly scalable applications, such online gaming platforms, chat apps, and real-time web applications.
- non-blocking, I/O operations: Node.js’ non-blocking I/O operations enable it to handle several requests concurrently without becoming blocked, resulting in enhanced performance and responsiveness. As a result, Node.js apps can handle large amounts of traffic with minimum hardware resources, lowering infrastructure costs and improving overall user experience.
- seamless communication: Node.js uses the WebSocket protocol and packages such as Socket.IO to allow for real-time data exchange and bidirectional communication between the client and server. This capability is useful for designing interactive web apps, collaboration tools, and multiplayer online games that require real-time updates and notifications.
- npm (Node Package Manager): Node.js is known for its enormous ecosystem of modules and libraries, which are available via npm (Node Package Manager), the world’s largest package repository. With over a million packages covering a wide variety of functionalities, developers may use existing solutions to faster development, increase productivity, and effectively meet complicated requirements.
- integrates seamlessly with other technologies and frameworks: Node.js works seamlessly with other technologies and frameworks, including Express.js for web server development, React.js for user interface development, MongoDB for NoSQL database management, and Docker for containerization, allowing developers to create comprehensive solutions using best-in-class tools and technologies.
- rapid prototyping and iteration: Node.js’ lightweight runtime and development tools, such as Nodemon for automatic server restarts, Debugger for debugging programs, and PM2 for process management, make quick prototyping and iteration possible. This agility allows developers to rapidly experiment, iterate, and release changes, decreasing time-to-market and facilitating continuous delivery processes.
Wrapping Up:
Node.js’s ease of handling asynchronous, event-driven programming has made it a mainstay in contemporary web development. It is possible to recognize Node.js’ true place in the development ecosystem by realizing that it is a runtime environment rather than a programming language or framework. Because of its high performance guaranteed by the V8 JavaScript engine, it’s a great option for developing scalable, quick network applications.
To sum up, Node.js is an effective solution that has extended JavaScript’s functionality from the browser to the server. Whether you’re creating real-time apps, web servers, or APIs, Node.js provides a stable and effective development environment to suit your demands.
Please feel free to contact with me on professional networks such as GitHub and LinkedIn if you’re interested in learning more about Node.js and keeping up with the newest trends and advances in web development. For updates on my projects and industry insights, you can also follow me on Instagram. Together, let’s stay in touch and advance in the fascinating field of technology.
THANKYOU 😊